GuidesDatabase
Table of Contents:
MongoDB
Setup
- Create a new project and deploy a cluster on MongoDB Atlas
- In your project on MongoDB Altas, click [Network Access] then [+ Add IP Address]. Enter
0.0.0.0/0
in [Access List Entry]. This allows connections from your computer and your production deployment(s) (Vercel for instance). - If you haven't done it yet, rename
.env.example
to.env.local
. Then add your connection string toMONGODB_URI
in.env.local
.
Run a local database for your dev setup so you can work offline and it's faster. You can read more here.
Mongoose (Optional)
Mongoose makes it easier to deal with MongoDB and has some cool features.
Models are defined in the folder /models
. Add any new models there.
The plugin toJSON is added to all models to remove the _id
and __v
(easier on front-end). Also if you add private: true
to any field it will be removed from the response. I.e. make email private so it's not sent to the front-end.
MongoServerSelectionError
Change the mongo.ts
file to:
mongo.ts
1import { MongoClient } from "mongodb";
2
3// This lib is use just to connect to the database in next-auth.
4// We don't use it anywhere else in the API routes—we use mongoose.ts instead (to be able to use models)
5// See /libs/next-auth.ts file.
6
7const uri = process.env.MONGODB_URI;
8const options = {};
9
10let client: MongoClient;
11
12if (!uri) {
13 console.group("⚠️ MONGODB_URI missing from .env");
14 console.error(
15 "It's not mandatory but a database is required for Magic Links."
16 );
17 console.error(
18 "If you don't need it, remove the code from /libs/next-auth.js (see connectMongo())"
19 );
20 console.groupEnd();
21} else if (process.env.NODE_ENV === "development") {
22 let globalWithMongo = global as typeof globalThis & {
23 _mongoClient?: MongoClient;
24 };
25
26 if (!globalWithMongo._mongoClient) {
27 globalWithMongo._mongoClient = new MongoClient(uri, options);
28 }
29 client = globalWithMongo._mongoClient;
30} else {
31 client = new MongoClient(uri, options);
32}
33export default client;
Supabase
Setup
- In Supabase SQL Editor, run this query to add a
profiles
table (an extension of the authenticated user to store data like Stripecustomer_id
, subscription access, etc...): - Go to the new
profiles
table and add 2 RLS policies: - Enable read access for authenticated users only
- Enable insert access for authenticated users only
- (Optional) If you want to collect leads with ButtonLead, create a new table called
leads
and add a RLS policy with insert access for anyone:
Supabase SQL Editor
1create table public.profiles (
2 id uuid not null references auth.users on delete cascade,
3 customer_id text,
4 price_id text,
5 has_access boolean,
6 email text,
7
8 primary key (id)
9);
10
11alter table public.profiles enable row level security;
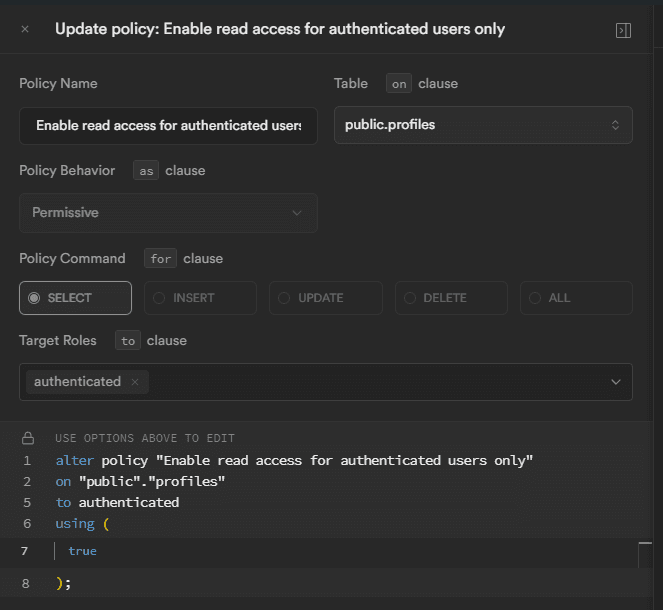
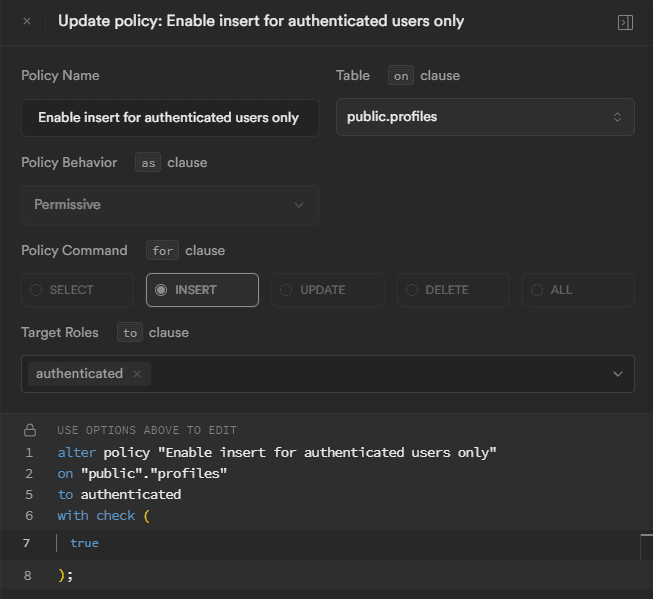
Supabase SQL Editor
1create table public.leads (
2 id uuid default gen_random_uuid(),
3 email text,
4 created_at timestamp with time zone default timezone('utc'::text, now()) not null,
5
6 primary key (id)
7);
8
9alter table public.leads enable row level security;